Hello, dear Python programming enthusiasts! Today, we'll talk about machine learning algorithms in Python. Machine learning might sound impressive, but it's like teaching computers to learn human thinking. Through vast amounts of data and algorithms, computers can learn and improve autonomously. Isn't that amazing? As a Python programmer, mastering these algorithms will undoubtedly elevate your skills. So, let's embark on this exciting learning journey!
Supervised Learning
Supervised learning is the most common type of algorithm in machine learning. Simply put, we give the algorithm a bunch of labeled data, allowing it to learn how to predict labels based on features. It's like a teacher (us) giving students (algorithms) a set of exercises and answers, teaching them how to solve problems. Doesn't it sound intuitive?
Linear Regression
Linear regression is perhaps the most basic and simple supervised learning algorithm. It tries to find the best-fitting line among data points. Imagine it as a rubber band connecting all data points and finding the position where the band is most "comfortable."
Here's how to implement linear regression in Python:
from sklearn.linear_model import LinearRegression
import numpy as np
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 5, 4, 5])
model = LinearRegression()
model.fit(X, y)
print(model.predict([[6]]))
This code looks simple, right? But the math behind it is quite complex. Don't worry, for now, we just need to know how to use it.
Did you know? Linear regression is used in many fields. For instance, real estate agents use it to predict house prices, marketers use it to predict sales, and even weather forecasts might employ linear regression!
Logistic Regression
Don't be misled by the name; logistic regression is actually used for classification. It's like the "brother" of linear regression, but its output is probability, typically used for binary classification problems.
Let's see how to implement logistic regression in Python:
from sklearn.linear_model import LogisticRegression
import numpy as np
X = np.array([[0, 0], [1, 1], [1, 0], [0, 1]])
y = np.array([0, 1, 1, 0])
model = LogisticRegression()
model.fit(X, y)
print(model.predict([[2, 2]]))
Logistic regression is very useful in many scenarios. For example, banks can use it to predict if someone will repay a loan, and doctors can use it to predict if a patient has a certain disease. Can you think of other applications?
K-Nearest Neighbors
The K-Nearest Neighbors (KNN) algorithm is very intuitive. Its idea is "birds of a feather flock together." Specifically, it finds the K nearest neighbors to the point to be classified and classifies it based on the "majority rule."
Let's see how to implement KNN in Python:
from sklearn.neighbors import KNeighborsClassifier
import numpy as np
X = np.array([[0, 0], [1, 1], [1, 0], [0, 1]])
y = np.array([0, 1, 1, 0])
model = KNeighborsClassifier(n_neighbors=3)
model.fit(X, y)
print(model.predict([[0.5, 0.5]]))
KNN is widely used in image recognition, recommendation systems, and more. For example, the music recommendation app you use probably employs the KNN algorithm!
Decision Trees
Decision trees are like a "20 questions" game. It reaches a conclusion through a series of yes/no questions. This algorithm is very intuitive and can even be visualized directly.
Let's see how to implement decision trees in Python:
from sklearn.tree import DecisionTreeClassifier
import numpy as np
X = np.array([[0, 0], [1, 1], [1, 0], [0, 1]])
y = np.array([0, 1, 1, 0])
model = DecisionTreeClassifier()
model.fit(X, y)
print(model.predict([[0.5, 0.5]]))
Decision trees are widely used in financial risk control, medical diagnosis, and more. For example, banks might use decision trees to decide whether to issue you a credit card. Interestingly, the thought process of decision trees is quite similar to humans, don't you think?
Unsupervised Learning
Compared to supervised learning, unsupervised learning is like self-learning without a teacher. The algorithm needs to discover patterns from unlabeled data. Does that sound harder? But don't worry, let's look at the most common unsupervised learning algorithms.
K-Means Clustering
The K-Means clustering algorithm is like playing a grouping game. It groups similar data points into clusters, eventually forming K groups (clusters). This algorithm is very useful in data analysis and customer segmentation.
Let's see how to implement K-Means clustering in Python:
from sklearn.cluster import KMeans
import numpy as np
X = np.array([[1, 2], [1, 4], [1, 0],
[4, 2], [4, 4], [4, 0]])
model = KMeans(n_clusters=2)
model.fit(X)
print(model.predict([[0, 0], [4, 4]]))
K-Means clustering is widely used in market segmentation, image compression, and more. For instance, you can use it to segment customers into different groups and develop targeted marketing strategies.
Algorithm Application and Practice
The purpose of learning these algorithms is, of course, to solve real-world problems. So, how can we apply these algorithms to practical work?
Data Preprocessing
Before applying any machine learning algorithm, data preprocessing is an essential step. This includes handling missing values, normalizing data, encoding categorical variables, and more.
For example, we can use the scikit-learn library to standardize data:
from sklearn.preprocessing import StandardScaler
import numpy as np
X = np.array([[1, 2], [3, 4], [5, 6]])
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
print(X_scaled)
Data preprocessing seems simple, but it has a significant impact on model performance. Did you know? Sometimes, good feature engineering is more important than choosing complex algorithms!
Model Evaluation and Optimization
After training the model, we need to evaluate its performance and optimize it. Common evaluation metrics include accuracy, precision, recall, etc. Optimization methods include cross-validation, grid search, and more.
Let's look at an example using cross-validation:
from sklearn.model_selection import cross_val_score
from sklearn.linear_model import LogisticRegression
import numpy as np
X = np.array([[0, 0], [1, 1], [1, 0], [0, 1]])
y = np.array([0, 1, 1, 0])
model = LogisticRegression()
scores = cross_val_score(model, X, y, cv=3)
print("Cross-validation scores:", scores)
print("Average score:", scores.mean())
Model evaluation and optimization is an iterative process. Sometimes, you might need to try various algorithms and adjust multiple parameters to get a satisfactory model. But don't be discouraged; this process, though challenging, is also filled with fun!
Real Case Analysis
Let's look at a real case: predicting house prices. This is a classic regression problem, and we can use linear regression to solve it.
First, we need to prepare the data:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error, r2_score
data = pd.read_csv('house_prices.csv')
X = data[['area', 'bedrooms', 'age']]
y = data['price']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = LinearRegression()
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
r2 = r2_score(y_test, y_pred)
print("Mean squared error: ", mse)
print("R-squared score: ", r2)
This example demonstrates a complete machine learning workflow: from data preparation to model training to model evaluation. You'll find that this process relates to all the parts we've learned before.
Conclusion
Well, dear readers, our journey through Python machine learning algorithms has come to an end. We've learned about several common supervised and unsupervised learning algorithms and explored how to apply these algorithms to real-world problems.
Did you notice that machine learning isn't that scary? It's like a powerful toolbox, and you are the craftsman who skillfully uses these tools.
Remember, the best way to learn machine learning is through practice. Try solving problems around you with these algorithms, and you'll find that the world can be interpreted in such an interesting way.
Are you ready to start your machine learning journey? Trust me; it will be a challenging yet fun-filled adventure. Let's explore this fascinating field together!
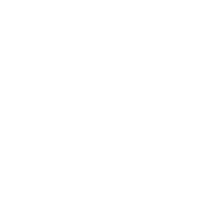
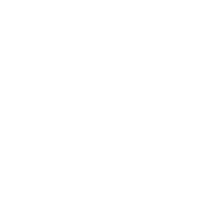