Hello, dear Python enthusiasts! Today we're going to explore a topic that's both magical and practical—algorithms in Python. Do you think the word "algorithm" sounds grand and hard to understand? Don't worry, follow me, and I promise you'll fall in love with the charm of algorithms.
What is an Algorithm
First, we need to figure out what exactly an algorithm is. Simply put, an algorithm is a series of steps to solve a problem. You can think of it as a recipe—telling you what to do step by step, ultimately resulting in a delicious outcome. In programming, an algorithm is a set of instructions that tells a computer how to solve a problem step by step.
So why do we learn algorithms? Imagine you need to find a specific book among a pile of books; how would you do it? Would you flip through them one by one? That's a simple "algorithm." But if the books are arranged alphabetically, you might use binary search to find the target faster. See, that's the magic of algorithms—they help us solve problems more efficiently.
Basics of Algorithms in Python
To implement algorithms in Python, we need to follow some basic principles:
-
Clarity: Code should be easy to understand. Remember, code is written for people to read and for machines to execute.
-
Efficiency: Minimize unnecessary calculations. Like cooking, we want to make the most delicious dishes with the least time and ingredients.
-
Scalability: Code should handle inputs of different sizes.
-
Robustness: Handle various possible inputs, including edge cases and exceptions.
Let's look at some common types of algorithms and see how they are implemented in Python.
Search Algorithms: The Art of Finding Treasure
Imagine you're playing a treasure hunt game; how would you search? That's what search algorithms aim to solve.
Linear Search: The Most Naive Method
Linear search is like looking for treasure room by room. Simple, but slow when there are many rooms. Check out this implementation:
def linear_search(lst, target):
for i in range(len(lst)):
if lst[i] == target:
return i
return -1
my_list = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
result = linear_search(my_list, 6)
print(f"The index of 6 in the list is: {result}")
This algorithm is intuitive, right? But if the list is long, it becomes slow. Is there a faster way?
Binary Search: The Smart Choice
If you've played the number guessing game, you might have used binary search. Guess the middle number each time, then narrow the range. That's the essence of binary search.
def binary_search(lst, target):
left, right = 0, len(lst) - 1
while left <= right:
mid = (left + right) // 2
if lst[mid] == target:
return mid
elif lst[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
sorted_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
result = binary_search(sorted_list, 7)
print(f"The index of 7 in the sorted list is: {result}")
Binary search is much faster than linear search, but it requires the list to be sorted. That's the trade-off in algorithms—gain some, lose some.
Hash Search: Direct to the Target
Hash search is like giving each element a unique "address," so you can go directly to that "address" to find the element. It's usually very fast.
def hash_search(lst, target):
hash_table = {}
for i, value in enumerate(lst):
hash_table[value] = i
return hash_table.get(target, -1)
my_list = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
result = hash_search(my_list, 9)
print(f"The index of 9 in the list is: {result}")
Hash search is extremely fast, but it requires extra space to store the hash table. Again, a trade-off—we trade space for time.
Sorting Algorithms: Bringing Order to Chaos
Sorting algorithms are like organizing your bookshelf. There are many ways to sort, each with its pros and cons.
Bubble Sort: Simple but Not Smart
Bubble sort is like letting bubbles rise to the surface, with larger bubbles rising first.
def bubble_sort(lst):
n = len(lst)
for i in range(n):
for j in range(0, n-i-1):
if lst[j] > lst[j+1]:
lst[j], lst[j+1] = lst[j+1], lst[j]
return lst
unsorted_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = bubble_sort(unsorted_list.copy())
print(f"Sorted list: {sorted_list}")
Bubble sort is easy to understand but not efficient for large lists. However, it's useful in specific cases, like when the list is almost sorted.
Quick Sort: Divide and Conquer
Quick sort is a more efficient sorting algorithm based on "divide and conquer."
def quick_sort(lst):
if len(lst) <= 1:
return lst
pivot = lst[len(lst) // 2]
left = [x for x in lst if x < pivot]
middle = [x for x in lst if x == pivot]
right = [x for x in lst if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
unsorted_list = [3, 6, 8, 10, 1, 2, 1]
sorted_list = quick_sort(unsorted_list)
print(f"Quick sorted list: {sorted_list}")
Quick sort is fast in most cases, but in the worst case (like when the list is already sorted), its efficiency drops. That's why we need to understand different sorting algorithms to choose the most suitable one for different situations.
Recursion: The Art of Algorithms
Recursion is like nesting dolls, a function repeatedly calling itself until it meets a condition to stop. It allows us to solve complex problems with concise code.
Fibonacci Sequence: The Magical Sequence of Nature
The Fibonacci sequence is common in nature, like the arrangement of sunflower seeds. Let's see how to implement it using recursion:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
for i in range(10):
print(f"The {i}th Fibonacci number is: {fibonacci(i)}")
This implementation is elegant, right? But it's not efficient because it repeats calculations many times. This is a common issue with recursion—conciseness but potentially low efficiency.
Factorial: A Basic Concept in Mathematics
Factorial is another concept that can be elegantly implemented with recursion:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
result = factorial(5)
print(f"The factorial of 5 is: {result}")
The beauty of recursion is that it breaks complex problems into simple sub-problems. But be careful, deep recursion can lead to stack overflow.
Dynamic Programming: The Algorithm of the Clever
Dynamic programming is a method for solving complex problems by breaking them down into simpler sub-problems. Its core idea is to remember the answers to solved sub-problems to avoid repeated calculations.
Fibonacci Sequence: Dynamic Programming Version
Remember the Fibonacci sequence we implemented with recursion? Let's optimize it with dynamic programming:
def fibonacci_dp(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
result = fibonacci_dp(100)
print(f"The 100th Fibonacci number is: {result}")
See the difference? This version is much faster because it avoids repeated calculations. That's the magic of dynamic programming!
Knapsack Problem: A Classic Dynamic Programming Problem
The knapsack problem is a classic dynamic programming problem. Imagine you're a thief (just a metaphor), with a limited-capacity bag, trying to steal the most valuable items in limited time.
def knapsack(weights, values, capacity):
n = len(weights)
dp = [[0 for _ in range(capacity + 1)] for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(1, capacity + 1):
if weights[i-1] <= w:
dp[i][w] = max(values[i-1] + dp[i-1][w-weights[i-1]], dp[i-1][w])
else:
dp[i][w] = dp[i-1][w]
return dp[n][capacity]
weights = [10, 20, 30]
values = [60, 100, 120]
capacity = 50
max_value = knapsack(weights, values, capacity)
print(f"The maximum value the knapsack can hold is: {max_value}")
This problem seems simple but involves many complex decisions. Dynamic programming helps us break this complex problem into simple sub-problems and then gradually build the final solution.
Greedy Algorithms: The Impulsive Choice
Greedy algorithms are like an impulsive person who chooses the best-looking option at each step without considering the long-term impact. Sometimes this strategy yields the optimal solution, sometimes it doesn't.
Coin Change Problem
Imagine you're a cashier needing to make change with the fewest coins. How would a greedy algorithm approach this?
def coin_change_greedy(coins, amount):
coins.sort(reverse=True) # Sort in descending order
count = 0
for coin in coins:
while amount >= coin:
amount -= coin
count += 1
return count if amount == 0 else -1
coins = [25, 10, 5, 1] # Cents
amount = 63
result = coin_change_greedy(coins, amount)
print(f"The minimum number of coins needed is: {result}")
This algorithm gives the optimal solution in the US coin system, but it might fail in some coin systems. That's the nature of greedy algorithms—simple and fast but not always optimal.
Activity Selection Problem
Suppose you're a conference room manager with many meeting requests, and you want to schedule as many meetings as possible. How would a greedy algorithm approach this?
def activity_selection(start, end):
n = len(start)
# Sort by end time
activities = sorted(zip(start, end), key=lambda x: x[1])
selected = [activities[0]]
last_end = activities[0][1]
for i in range(1, n):
if activities[i][0] >= last_end:
selected.append(activities[i])
last_end = activities[i][1]
return selected
start = [1, 3, 0, 5, 8, 5]
end = [2, 4, 6, 7, 9, 9]
result = activity_selection(start, end)
print(f"The selected activities are: {result}")
This greedy strategy—always choosing the next earliest ending activity—actually yields the optimal solution for this problem. That's the charm of greedy algorithms: in some problems, they can provide the optimal solution in the simplest way.
Applications of Algorithms: From Theory to Practice
After discussing so much theory, you might ask: how are these algorithms used in practice? Let me give you some examples:
-
Search Engines: When you search on Google, efficient search algorithms are at work behind the scenes.
-
Navigation Systems: When you use navigation software, it's using graph algorithms to find the shortest path.
-
Recommendation Systems: Netflix or Amazon's recommendation features are powered by complex machine learning algorithms.
-
Image Processing: Instagram's filter functions use various image processing algorithms.
-
Financial Trading: High-frequency trading employs complex algorithms to make quick decisions.
These are just the tip of the iceberg. In reality, algorithms are everywhere, quietly changing our lives.
Algorithm Optimization: Making Your Code Fly
Now that we know these algorithms, the next step is how to make them run faster. Here are a few tips:
-
Choose the Right Data Structure: For frequent lookup operations, using a dictionary (hash table) might be better than a list.
-
Avoid Repeated Calculations: Use caching or dynamic programming to store intermediate results.
-
Use Built-in Functions: Python's built-in functions are usually faster than custom loops.
-
Consider Space-Time Trade-offs: Sometimes, we can sacrifice some memory to gain speed.
-
Use Performance Analysis Tools: Python's cProfile module can help identify bottlenecks in your code.
Remember, premature optimization is the root of all evil. Get the code running correctly first, then think about how to make it faster.
Conclusion: The Charm of Algorithms
Our journey into algorithms ends here. Have you felt the charm of algorithms? They are like magic spells for solving problems, making complex problems simple and slow programs fast.
But remember: no algorithm is a panacea. Each algorithm has its applicable scenarios and limitations. As a good programmer, our task is to choose the most suitable algorithm for different situations.
Finally, I want to say: learning algorithms is not just about writing better code, but also about developing a problem-solving mindset. When you master these ways of thinking, you'll find that not only programming problems but many life problems can be solved similarly.
So, are you ready to continue this wonderful journey of algorithms? Remember, practice makes perfect. Try implementing these algorithms, solve problems on LeetCode, apply them in real projects. Trust me, once you truly master these algorithms, you'll feel like you have superpowers!
Which algorithm are you most interested in? Is there anything you don't understand? Or are there other algorithms you want to learn about? Feel free to leave a comment, let's discuss and grow together!
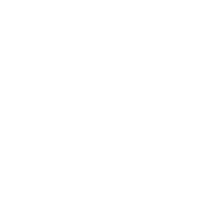
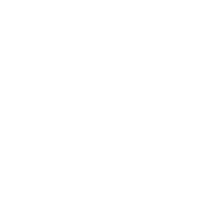