Hello, dear Python enthusiasts! Today we're going to talk about advanced algorithm knowledge in Python. I believe you already have some understanding of basic algorithm concepts, so let's challenge ourselves and explore some more advanced algorithmic ideas. We'll start with recursion and gradually delve into dynamic programming, examining how these algorithms make our code more efficient and elegant. Ready? Let's embark on this exciting algorithm journey!
The Beauty of Recursion
Recursion—doesn't it sound a bit mysterious? Actually, it's not. Its core idea is very simple: a function calls itself. But this simple concept can solve many complex problems. Let's look at a classic recursion example—calculating factorial:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
print(factorial(5)) # Output: 120
Isn't this code amazing? The function calls itself internally! That's the charm of recursion. It breaks down a big problem into smaller, similar problems and solves them step by step.
However, note that while recursion is elegant, it's not always the optimal solution. For example, when calculating the Fibonacci sequence, simple recursion may lead to a lot of repeated calculations. That's when we need to consider using more advanced algorithmic strategies.
Divide and Conquer
Speaking of advanced algorithmic strategies, we must mention "divide and conquer." As the name suggests, it involves breaking a complex problem into two or more identical or similar subproblems until the subproblems can be solved directly. The solution to the original problem is the combination of the solutions to the subproblems.
Let's look at a classic divide-and-conquer algorithm—merge sort:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
arr = [38, 27, 43, 3, 9, 82, 10]
sorted_arr = merge_sort(arr)
print(sorted_arr) # Output: [3, 9, 10, 27, 38, 43, 82]
Does this algorithm seem a bit complex? Don't worry, let's understand it step by step. The core idea of merge sort is: first, divide the array into two halves, sort them separately, and then merge them. This process recurses until the subarray length is 1. Finally, we merge these sorted small arrays into a large sorted array.
The advantage of divide and conquer is that it can transform a complex problem into several smaller, identical problems, which are often easier to solve. Moreover, divide and conquer can often effectively use parallel processing to improve efficiency.
Greedy Algorithm
Next, let's look at another interesting algorithmic strategy—the greedy algorithm. The core idea of the greedy algorithm is: in each step, make the best or optimal choice in the current state, hoping to lead to the best or optimal result.
Sounds simple, right? But note, greedy algorithms do not always yield a globally optimal solution. They are more of a heuristic that can quickly provide a good solution for some problems.
Let's look at a classic greedy algorithm problem—making change:
def coin_change(coins, amount):
coins.sort(reverse=True) # Sort from largest to smallest
count = 0
for coin in coins:
while amount >= coin:
amount -= coin
count += 1
return count if amount == 0 else -1
coins = [25, 10, 5, 1] # Coin denominations
amount = 63 # Amount to make change for
result = coin_change(coins, amount)
print(f"Number of coins needed: {result}") # Output: Number of coins needed: 6
The idea of this algorithm is: always use the largest denomination coin first. This works in the US currency system because the coin denominations are 25 cents, 10 cents, 5 cents, and 1 cent, which are multiples of each other. However, if the coin system is not designed this way, the greedy algorithm may not yield the optimal solution.
Backtracking
After discussing greedy algorithms, let's look at another powerful algorithmic strategy—backtracking. Backtracking is essentially a trial-and-error method. Its core idea is: move forward on a path, proceed if possible, retreat if not, and try another path.
Sounds like the way we solve mazes, right? Exactly, backtracking is a method that explores all possibilities to find the correct answer.
Let's look at a classic backtracking problem—generating parentheses:
def generate_parenthesis(n):
def backtrack(S, left, right):
if len(S) == 2 * n:
result.append(''.join(S))
return
if left < n:
S.append('(')
backtrack(S, left+1, right)
S.pop()
if right < left:
S.append(')')
backtrack(S, left, right+1)
S.pop()
result = []
backtrack([], 0, 0)
return result
n = 3
print(generate_parenthesis(n))
This problem requires generating all possible valid combinations of parentheses. We use backtracking, where each time we can choose to add a left or right parenthesis, but we must follow certain rules: the number of left parentheses cannot exceed n
, and the number of right parentheses cannot exceed the number of left parentheses.
The advantage of backtracking is that it can systematically search all possible solutions and prune invalid paths during the search, avoiding unnecessary computations.
Dynamic Programming
Finally, we've reached the "big boss" of algorithms—dynamic programming. The core idea of dynamic programming is to decompose a complex problem into several subproblems and solve the complex problem by solving the subproblems. It is similar to divide and conquer, but dynamic programming saves the solutions to subproblems to avoid repeated calculations.
Let's look at a classic dynamic programming problem—climbing stairs:
def climb_stairs(n):
if n <= 2:
return n
dp = [0] * (n + 1)
dp[1] = 1
dp[2] = 2
for i in range(3, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
n = 5
print(f"There are {climb_stairs(n)} ways to climb {n} stairs")
The problem is: suppose you are climbing stairs, and it takes n
steps to reach the top. Each time you can climb 1 or 2 steps. How many different ways can you climb to the top?
The dynamic programming idea is that the number of ways to reach the i
-th step is equal to the number of ways to reach the (i-1)
-th step plus the number of ways to reach the (i-2)
-th step. This is because you can reach i
by climbing 1 step from (i-1)
or 2 steps from (i-2)
.
The advantage of dynamic programming is that it can effectively solve problems with overlapping subproblems and optimal substructure properties. By storing intermediate results, dynamic programming greatly reduces computation and improves algorithm efficiency.
Conclusion
Well, our algorithm journey ends here. From recursion to dynamic programming, we've seen the gradual deepening and sublimation of algorithmic ideas. Each algorithm has its characteristics and applicable scenarios:
- Recursion is suitable for solving problems with recursive relationships, offering concise and elegant code but may involve repeated calculations.
- Divide and conquer decomposes a big problem into smaller ones, suitable for problems with divide-and-conquer characteristics, like sorting and searching.
- Greedy algorithms make the best choice at each step, suitable for some optimization problems but may not yield a globally optimal solution.
- Backtracking searches for all possible solutions through trial and error, suitable for permutation and combination problems.
- Dynamic programming improves efficiency by saving subproblem solutions, suitable for problems with overlapping subproblems and optimal substructure.
Which of these algorithms attracts you the most? Each one has its unique charm. The key is to choose the appropriate algorithm based on the specific problem. In real-world programming, we often need to use multiple algorithmic strategies together to solve complex problems.
I hope this article helps you better understand these advanced algorithmic strategies. Remember, learning algorithms is a gradual process that requires a lot of practice and thought. So, start practicing! Try using these algorithms to solve real problems, and I'm sure you'll gain deeper insights.
Lastly, do you have any thoughts or questions? Feel free to leave a comment, and let's explore the mysteries of Python algorithms together!
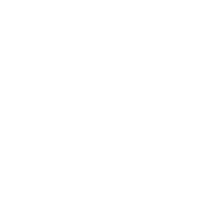
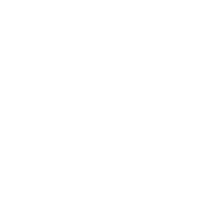
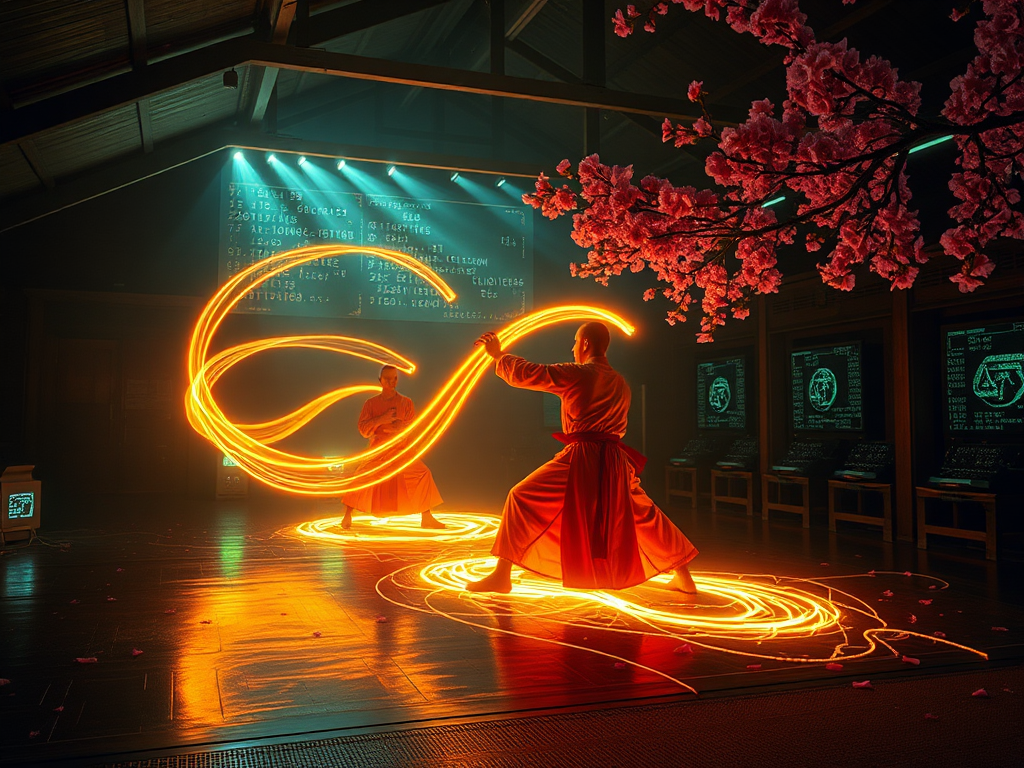
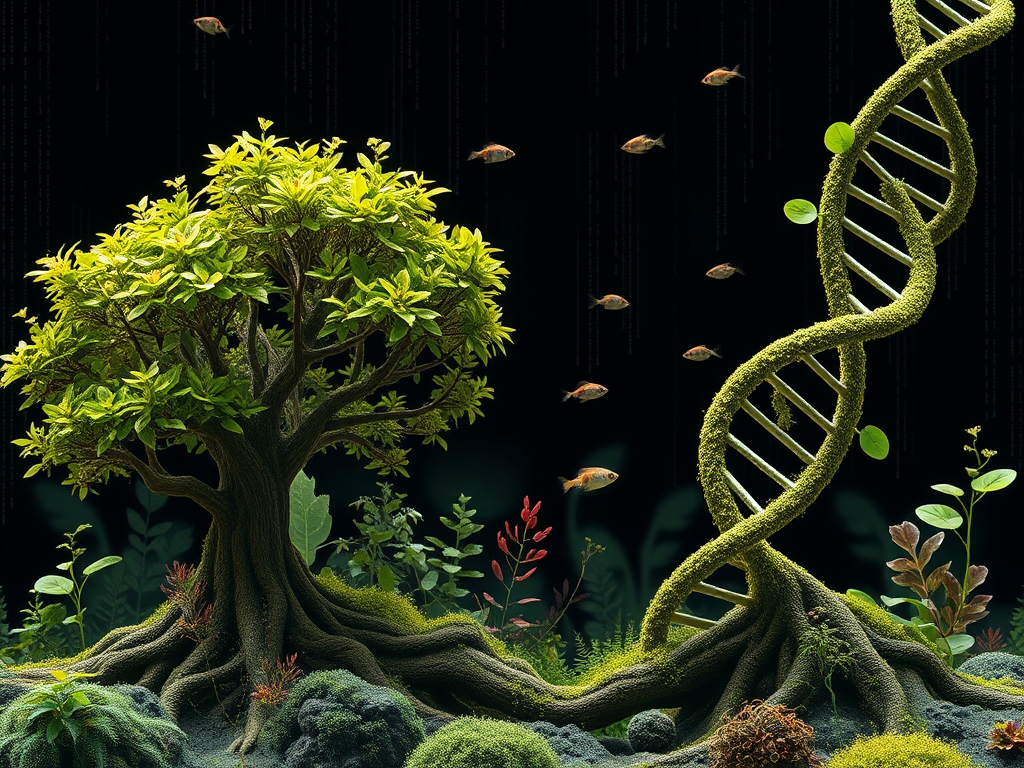
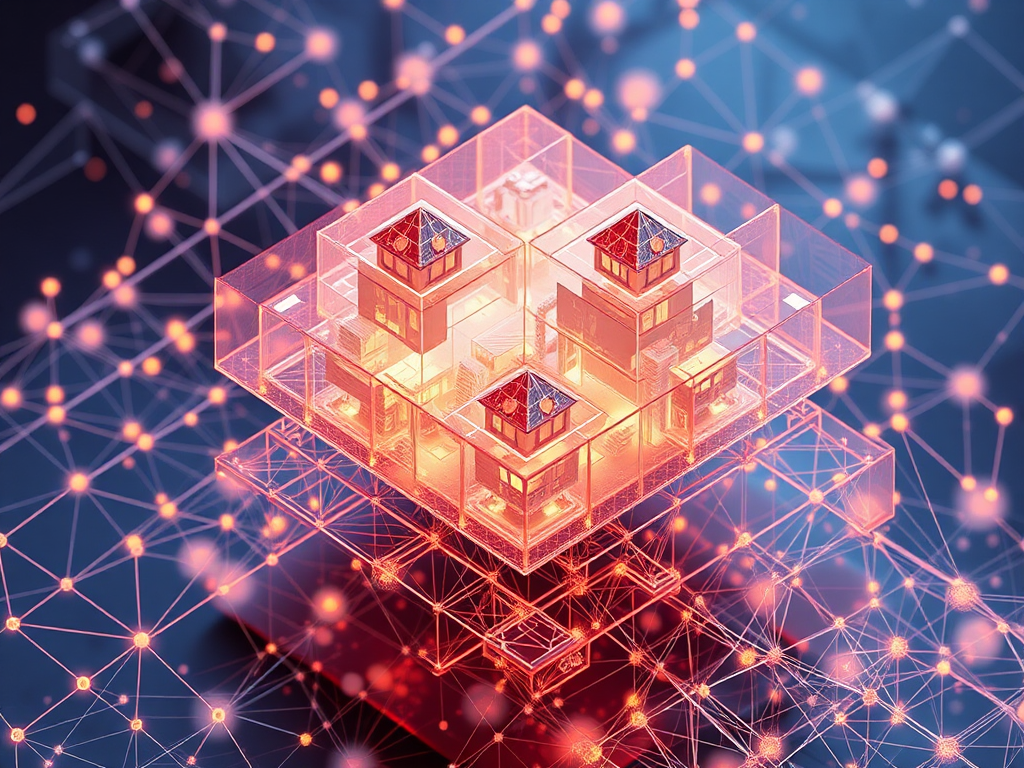