Hello everyone, today we're going to discuss some advanced applications and optimization techniques for Python algorithms. Did you know that mastering efficient algorithms can not only make your programs run at lightning speed but also give you a significant edge in interviews?
The Magical Trick of Merge Sort
Let's start with the classic merge sort algorithm. Besides efficient sorting, it has a surprising magical use—finding the number of inversions in an array! How do we do this? Let's break it down step by step:
- First, divide the array in half and find the number of inversions in each subarray
- While merging the two sorted subarrays, traverse both arrays and count inversions whenever encountered
- Finally, the sum of inversions in the subarrays is the total number of inversions in the entire array!
See, by cleverly utilizing the merge sort approach, we can efficiently solve this seemingly complex problem in O(nlogn) time complexity. This technique of applying existing algorithmic ideas to new problems is a common ace up the sleeve in algorithm design.
Dynamic Programming Shows Its Power
Now let's look at the powerful tool of dynamic programming, which can help us easily solve many complex problems. For instance, consider the classic hiking problem: suppose you're going on a hike and will encounter different risk values along the way. How should you walk to minimize the total risk value?
We can think about it like this:
- Define dp[i] as the minimum risk value from the starting point to the i-th point
- The state transition equation is: dp[i] = min(dp[i-1], dp[i-2], ...) + risk[i]
- Through dynamic programming, we can find the final minimum risk value!
See, breaking down complex problems into simple repeating subproblems and solving them through state transition equations is the charm of dynamic programming. Once you master it, you'll be able to tackle various difficult problems with ease.
Advanced Applications of Network Flow Algorithms
Next, let's look at the application of network flow algorithms in real-world problems. For example, consider this scenario: if the flows of two edges are variables and their total flow has an upper limit, how do we calculate the maximum flow of the entire network?
In this case, we can use the binary search method:
- First, binary search the range of upper and lower bounds of the total flow
- For each middle value, try to find the optimal combination of flows for the two edges while satisfying the total flow constraint
- If feasible, continue to increase the lower bound; otherwise, decrease the upper bound
- The final middle value is the maximum flow that satisfies the total flow constraint!
See, by combining binary search and network flow algorithms, we can elegantly solve this constrained maximum flow problem. Isn't it interesting to integrate multiple algorithmic ideas?
The Power of Data Structures and Parallel Computing
Finally, let's look at how to improve the execution efficiency of some algorithms. For instance, to speed up a ray tracing algorithm based on analytical methods, we can do the following:
- Optimize data structures, such as using K-D trees to accelerate ray-object intersection tests
- Parallel computing, utilizing the parallel capabilities of multi-core CPUs or GPUs to process multiple rays simultaneously
See, by properly leveraging the power of data structures and parallel computing, we can greatly enhance the execution efficiency of algorithms. This can be the solution for problems with massive computational requirements.
In conclusion, I hope you've picked up some techniques for algorithm design and optimization from today's sharing. Remember, algorithms are not just popular knowledge points for exams, but also essential foundational skills for programmers. Master algorithms well, and you'll excel in both programming practice and job interviews!
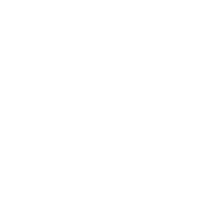
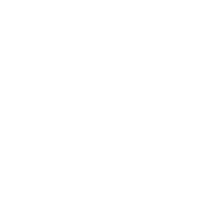