Hello everyone! Today we'll discuss some key aspects to focus on in Python secure programming. As Python programmers, we should not only pursue code efficiency and practicality but also pay attention to code security. After all, once code has security vulnerabilities, it can not only cause economic losses to businesses but also lead to serious data breaches. So, let's learn together how to write more secure Python programs!
Code Protection
As Python programmers, the code we write is like our "intellectual property" and needs to be properly protected. If the code is stolen or pilfered by malicious individuals, that would be terrible. So we need to find ways to protect our code and prevent it from easily falling into the hands of others.
Code Obfuscation
Have you heard of the technique called "code obfuscation"? Its purpose is to make the code more obscure and difficult to understand, preventing others from stealing your code. We can use tools like pyarmor
or Cython
to obfuscate the code. This way, even if someone gets hold of your code, it will be difficult for them to understand its true meaning.
However, when using code obfuscation techniques, we should also be careful not to over-obfuscate to the point of affecting code readability. After all, we or our team members will need to maintain this code in the future. So, moderate code obfuscation is key.
Compiling to Binary
In addition to code obfuscation, we can also compile Python code into binary files. This way, others cannot directly view the source code. We can use tools like PyInstaller
or cx_Freeze
to achieve this.
However, this method also has a drawback, which is that it increases the size of the program. So, when deciding whether to compile code into binary, we need to weigh the pros and cons.
License Management
Finally, we can implement a license management system to limit the use and distribution range of the software. This way, even if someone obtains your code, they cannot use it without authorization.
Of course, implementing a license management system requires some cost and effort. But if your code is very important and has commercial value, then this approach is quite necessary.
Data Security
In addition to protecting the code itself, we also need to focus on data security. After all, data is a very valuable resource for most applications. If data is compromised or leaked, it would be a big problem.
Secure Password Storage
Let's first talk about secure password storage. As a programmer, you must know that storing passwords in plain text in a database is a very dangerous practice. Because once the database is compromised, all users' passwords will be exposed.
So, the correct approach is to use a hashing algorithm to store passwords. We recommend using strong hashing algorithms like bcrypt
or argon2
, which have high security. At the same time, we should add a random "salt" before hashing the password to prevent rainbow table attacks.
In addition, we should regularly update the hashing algorithm and salt used to enhance security. If you find these operations too cumbersome, you can also use some ready-made libraries, such as passlib
, which can greatly simplify the process of password storage and verification.
You see, protecting user password security is not actually difficult. As long as we are careful and take necessary measures, we can avoid many potential security risks.
Input Validation
Of course, in addition to passwords, we also need to pay attention to the security of other user inputs. Any untrusted input may have security risks, so we must strictly validate and sanitize all inputs to ensure that only data in the expected format is accepted.
The purpose of doing this is to prevent some common attack methods, such as SQL injection attacks and XSS attacks. If we don't sufficiently check and filter user input, we are likely to be exploited by these attack methods, leading to data leaks or system intrusion.
Therefore, input validation is an important part of ensuring data security. When writing code, we need to be extra careful and strictly check every user input, and never be careless.
Using Parameterized Queries
We mentioned SQL injection attacks above, which is a very common and dangerous attack method. Its principle is to inject malicious code into SQL query statements to obtain or modify data in the database.
To prevent SQL injection attacks, we need to use parameterized queries. This query method can separate the query statement and parameters, thus avoiding the risk of directly concatenating user input into SQL statements.
For example, suppose we need to query user information based on user ID, we can write like this:
user_id = input("Please enter user ID: ")
cursor.execute("SELECT * FROM users WHERE id = %s", (user_id,))
This way, no matter what the user inputs, it will not affect the structure and semantics of the SQL statement, thus effectively preventing SQL injection attacks.
In addition to using parameterized queries, we can also use ORM (Object-Relational Mapping) frameworks, such as SQLAlchemy or Django ORM. These frameworks have built-in protection against SQL injection, which can greatly reduce our manual protection work.
API Security
With the rise of microservice architecture and cloud computing, APIs (Application Programming Interfaces) play an increasingly important role in modern applications. However, APIs also have some security risks, so we must pay attention to API security.
Authentication and Authorization
When designing APIs, we need to implement authentication and authorization mechanisms to ensure that only authorized users can access the corresponding resources and functions. Common authentication methods include OAuth2 and JWT (JSON Web Tokens).
Using these standardized authentication protocols not only improves the security of the system but also enhances the scalability and maintainability of the system. After all, we don't want to reinvent the wheel ourselves, do we?
HTTPS Communication
Another very important aspect is that we must ensure that all API communications are conducted through HTTPS. HTTPS can provide encryption protection for data transmission, preventing data from being stolen or tampered with during transmission.
In modern web applications, HTTPS has become a basic security requirement. If your API is still using HTTP for communication, that's really out of date. So, please switch to HTTPS as soon as possible.
Rate Limiting
Finally, we need to implement a rate limiting mechanism to prevent DDoS attacks and API abuse. Rate limiting can limit the number of API accesses per user or IP address within a certain time, thus avoiding excessive consumption of system resources.
Of course, the specific limitation strategy needs to be formulated according to the actual situation. We should neither set it too loose nor too strict. Only reasonable limitations can truly be effective.
Web Application Security
In addition to API security, we also need to pay attention to the overall security of web applications. After all, many Python applications provide services in web form.
Flask Application Security Configuration
Taking the Flask framework as an example, we need to pay attention to some security configuration options. For instance, we should use the Flask-Login
extension to implement user authentication and session management, rather than manually handling these complex logics ourselves.
At the same time, we need to ensure that secure configuration options are used, such as setting a sufficiently complex SECRET_KEY
and turning off DEBUG
mode. These seemingly small configurations have a significant impact on the security of the application.
CSRF Protection
Another thing to note is CSRF (Cross-Site Request Forgery) attacks. This attack method can allow attackers to perform unexpected operations in the user's session, such as transferring money or changing passwords.
To prevent CSRF attacks, we can use the CSRF protection function provided by the Flask-WTF
extension. This extension can automatically generate and verify CSRF tokens, thus effectively preventing the occurrence of CSRF attacks.
XSS Defense
Finally, we should also pay attention to XSS (Cross-Site Scripting) defense. XSS attacks can allow attackers to inject malicious scripts into web pages, thereby obtaining users' sensitive information or performing other malicious operations.
To defend against XSS attacks, we need to strictly validate and sanitize all user inputs to ensure that no malicious script code is injected into the page. At the same time, we can also use some secure coding practices, such as escaping or encoding user input.
General Security Principles
In addition to the specific security measures mentioned above, we also need to follow some general security principles to ensure the overall security of the application.
Principle of Least Privilege
The principle of least privilege is a very important security principle. Its core idea is that each user or process is only granted the minimum privileges needed to perform specific tasks.
For example, in a database, we should create a separate account for each user or application and only grant it the minimum privileges it needs. This way, even if an account is compromised, it will only affect the data and functions that the account can access, thus minimizing the risk.
Secure Coding Practices
Another principle is that we must follow secure coding practices to avoid common security vulnerabilities. This includes but is not limited to SQL injection, XSS, CSRF, and other attack methods.
When writing code, we should always keep security in mind and guard against every potential vulnerability. At the same time, we should also continuously learn and update our security knowledge, as new attack methods and vulnerabilities will constantly emerge.
Regular Updates and Maintenance
Finally, we need to regularly update and maintain the application to fix known security vulnerabilities and address newly emerging threats.
There's a very important point here, which is that we can't stop at writing secure code, but need to continuously monitor the running status of the application and promptly discover and fix any security issues. Only in this way can our application truly achieve long-term security and reliability.
Summary
Alright, that's all for today. Through the above sharing, you should have a basic understanding of Python secure programming.
Security is an issue we need to prioritize when writing any application. Whether it's code protection, data security, or API and web application security, we need to take corresponding measures to ensure the overall security of the system.
At the same time, we also need to follow some general security principles, such as the principle of least privilege, secure coding practices, etc. Only in this way can our applications truly achieve long-term reliability and security.
Of course, security is an endless process. With the continuous emergence of new technologies and new threats, we need to continuously learn and update our security knowledge. But as long as we persist in our efforts, we can definitely write more secure and reliable Python applications.
So, do you have any other security concerns or questions? Welcome to continue the exchange and discussion in the comments section, let's work together to contribute to Python's security!
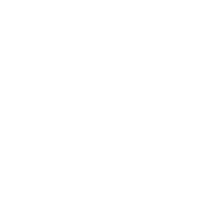