Hello, dear Python programming enthusiasts! Today we’re going to talk about a very practical topic—Python database operations. Whether you're a beginner just starting out or a developer with some experience, mastering database operations is an essential skill. So, let’s dive into this topic and see how to elegantly operate databases using Python!
Connecting to a Database
First, we need to learn how to connect to a database. It's like opening a door to the data world. Did you know? Python provides a great standard—the Python DB API, which allows us to connect to different types of databases in a unified way. Isn’t that thoughtful?
Let’s take MySQL as an example and see how to establish a connection:
import mysql.connector
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
cursor = connection.cursor()
cursor.execute("SELECT VERSION()")
version = cursor.fetchone()
print(f"Database version: {version[0]}")
cursor.close()
connection.close()
Looks simple, right? But these few lines of code contain many important concepts. First, we imported the mysql.connector
module. Then, we created a connection object, specifying the host, username, password, and database name. Next, we created a cursor object—like a pointer moving around in the database.
Did you notice that we closed the cursor and connection at the end of the code? This is a good habit that helps us release resources and avoid unnecessary issues.
Data Operations
Once connected to the database, we can start performing various operations. It’s like having a magical key to add, delete, update, and query data. Let’s take a look at these basic operations!
Creating a Table
First, we need a place to store data, right? That’s the role of creating a table. Check out the following code:
create_table_query = """
CREATE TABLE IF NOT EXISTS employees (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100),
age INT,
department VARCHAR(100)
)
"""
cursor.execute(create_table_query)
connection.commit()
This code creates a table named employees
. We defined fields like id, name, age, and department. Notice the IF NOT EXISTS
? It’s a small trick to avoid errors when creating a table that already exists.
Inserting Data
With a table, we can start adding data. It’s like putting things into a drawer:
insert_query = """
INSERT INTO employees (name, age, department)
VALUES (%s, %s, %s)
"""
employee_data = ("Zhang San", 28, "R&D")
cursor.execute(insert_query, employee_data)
connection.commit()
print(f"Successfully added {cursor.rowcount} record(s)")
Here we used parameterized queries, with %s
as placeholders. This not only makes the code clearer but also prevents SQL injection attacks. Safety first, right?
Querying Data
After inserting data, we definitely want to see the results. It’s like opening a drawer to check what we just put in:
select_query = "SELECT * FROM employees WHERE age > %s"
cursor.execute(select_query, (25,))
results = cursor.fetchall()
for row in results:
print(f"ID: {row[0]}, Name: {row[1]}, Age: {row[2]}, Department: {row[3]}")
This code queries all employees older than 25. The fetchall()
method returns all matching records. We can iterate through these records and print each employee’s information.
Updating Data
Sometimes, we need to modify existing data. For example, if an employee gets promoted, we need to update their department information:
update_query = "UPDATE employees SET department = %s WHERE name = %s"
new_data = ("HR", "Zhang San")
cursor.execute(update_query, new_data)
connection.commit()
print(f"Successfully updated {cursor.rowcount} record(s)")
This code updates Zhang San’s department to HR. The rowcount
attribute tells us how many records were updated.
Deleting Data
Finally, sometimes we need to delete data that’s no longer needed:
delete_query = "DELETE FROM employees WHERE age > %s"
cursor.execute(delete_query, (50,))
connection.commit()
print(f"Successfully deleted {cursor.rowcount} record(s)")
This example deletes all employee records over 50 years old. Of course, in real applications, we might use soft deletes instead of directly deleting data.
Exception Handling
When working with databases, we might encounter various unexpected situations, like network interruptions or insufficient permissions. This is where exception handling becomes crucial. Let’s see how to elegantly handle these situations:
try:
# Perform some database operations
cursor.execute(insert_query, employee_data)
connection.commit()
except mysql.connector.Error as err:
print(f"Oops! Something went wrong: {err}")
connection.rollback() # Roll back the transaction
finally:
cursor.close()
connection.close()
This code uses a try-except-finally
structure. If an error occurs, we print the error message and roll back the transaction. Whether or not an error occurs, we close the cursor and connection in the finally
block. This ensures resources are properly released.
Connection Pooling
If your application needs to connect to the database frequently, using a connection pool can greatly improve efficiency. A connection pool is like an administrator that manages and allocates database connections for us:
from mysql.connector import pooling
pool = pooling.MySQLConnectionPool(
pool_name="mypool",
pool_size=5,
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
connection = pool.get_connection()
try:
cursor = connection.cursor()
cursor.execute("SELECT * FROM employees")
for row in cursor:
print(row)
finally:
cursor.close()
connection.close() # Actually returns the connection to the pool
Using a connection pool reduces the overhead of frequently creating and destroying connections, improving application performance. Especially in high concurrency scenarios, the role of connection pooling becomes more apparent.
Transaction Management
When dealing with important data, we often need to use transactions to ensure data consistency. A transaction is like an indivisible operation group that either all succeed or all fail:
try:
connection.start_transaction()
cursor.execute("UPDATE accounts SET balance = balance - 100 WHERE id = 1")
cursor.execute("UPDATE accounts SET balance = balance + 100 WHERE id = 2")
connection.commit()
print("Transfer successful!")
except mysql.connector.Error as err:
connection.rollback()
print(f"Transfer failed: {err}")
In this example, we simulate a transfer operation. If any step goes wrong, the entire transfer will be canceled. This ensures our account data remains consistent.
Performance Optimization
When dealing with large amounts of data, performance becomes crucial. Here are a few tips to help you optimize database operations:
-
Use batch inserts:
python insert_query = "INSERT INTO employees (name, age, department) VALUES (%s, %s, %s)" employee_data = [ ("Li Si", 30, "Marketing"), ("Wang Wu", 35, "Finance"), ("Zhao Liu", 28, "Tech") ] cursor.executemany(insert_query, employee_data) connection.commit()
-
Use indexes wisely:
python cursor.execute("CREATE INDEX idx_name ON employees(name)")
-
Avoid using
SELECT *
, select only needed columns:python cursor.execute("SELECT name, age FROM employees WHERE department = 'Tech'")
-
Use EXPLAIN to analyze query performance:
python cursor.execute("EXPLAIN SELECT * FROM employees WHERE age > 30") print(cursor.fetchall())
These tips can help your queries run faster and more efficiently. Remember, optimization is an ongoing process that requires continuous testing and adjustment.
Security Considerations
When handling database operations, security is a topic that cannot be ignored. Here are some suggestions:
- Always use parameterized queries to prevent SQL injection: ```python # Good practice cursor.execute("SELECT * FROM users WHERE username = %s", (username,))
# Bad practice cursor.execute(f"SELECT * FROM users WHERE username = '{username}'") ```
-
Use the principle of least privilege, using different database users for different operations:
python read_only_connection = mysql.connector.connect( host='localhost', user='read_only_user', password='read_only_password', database='your_database' )
-
Encrypt sensitive data: ```python import hashlib
def hash_password(password): return hashlib.sha256(password.encode()).hexdigest()
cursor.execute("INSERT INTO users (username, password) VALUES (%s, %s)", (username, hash_password(password))) ```
- Regularly back up data: ```python import subprocess
def backup_database(): subprocess.run(["mysqldump", "-u", "username", "-p", "password", "database_name", ">", "backup.sql"])
# Regularly call the backup_database() function ```
Remember, security is not a one-time job but a process that requires continuous attention and improvement.
Conclusion
Well, dear readers, our Python database operations journey ends here. We started with the basics of connecting, explored adding, deleting, updating, querying, exception handling, connection pooling, transaction management, performance optimization, and even discussed security concerns. These knowledge points cover most of the scenarios you encounter in daily work.
Did you notice that database operations are like managing a huge warehouse? We need to know how to open the warehouse door (connect to the database), how to store things in the warehouse (insert data), how to find what we need (query data), how to update inventory information (update data), and how to handle items we no longer need (delete data).
In this process, we also need to pay attention to security (exception handling and security considerations), improve efficiency (connection pooling and performance optimization), and ensure all operations are reliable (transaction management). These skills are not only applicable to database operations but also a general approach to handling various problems in the programming world.
I hope this article helps you better understand Python database operations. Remember, programming is a practice-based art. So, don’t just stop at reading, try it out, practice, make mistakes, and learn from them. Only then can you truly master these skills.
Are you ready to start your Python database operations journey? If you have any thoughts or questions, feel free to leave a comment, and let’s discuss and grow together!
Happy coding, and see you next time!
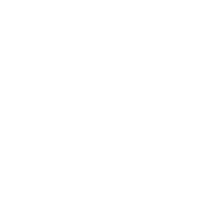