Have you ever felt frustrated while coding, as if you're reinventing the wheel? Don't worry, the Python Standard Library is here to solve that problem. Today, let's dive into the charm of the Python Standard Library and see how it can enhance your code.
What is the Standard Library
First, let's clarify what the Python Standard Library is. Simply put, it's a collection of pre-packaged modules that come installed with the Python interpreter, requiring no additional downloads. These modules cover everything from file operations to network programming, serving as a toolbox for Python programmers.
You might wonder, why use the Standard Library? Imagine if every time you needed to handle time, manipulate files, or generate random numbers, you had to code from scratch. That would be quite troublesome. The Standard Library provides a ready-made set of tools, allowing you to complete tasks quickly and efficiently.
Common Modules
Next, let's look at some commonly used standard library modules and see what they can do for us.
os Module: An Operating System Helper
The os module is a bridge to interact with the operating system. Whether it's creating folders, deleting files, or getting the current working directory, the os module makes it easy.
For example, to list all files in the current directory, you just need to do this:
import os
files = os.listdir('.')
print(files)
Simple, right? Here, '.' represents the current directory. You can also use os.path.join()
to construct cross-platform file paths, avoiding issues with different path separators across operating systems.
datetime Module: Time Handling Expert
When it comes to time handling, the datetime module is your go-to tool. Whether it's getting the current time or calculating date differences, it handles it with ease.
Want to know the current time? Try this:
from datetime import datetime
now = datetime.now()
print(f"It is now {now.hour}:{now.minute}")
You can even use timedelta
to perform time calculations, like finding out the date 100 days from now:
from datetime import datetime, timedelta
future = datetime.now() + timedelta(days=100)
print(f"100 days later it will be {future.month}/{future.day}")
random Module: Random Number Generator
Need to generate random numbers? The random module is made for this purpose. From simple random integers to complex probability distributions, it can handle it all.
Let's simulate a dice roll:
import random
dice = random.randint(1, 6)
print(f"You rolled a {dice}")
You can even use random.choice()
to randomly select an element from a list:
fruits = ['Apple', 'Banana', 'Orange', 'Grape']
print(f"Today, eat {random.choice(fruits)}")
json Module: Data Exchange Powerhouse
In modern web development, the JSON data format is ubiquitous. Python's json module lets us easily convert between Python objects and JSON strings.
For example, if you have a dictionary you want to convert to a JSON string:
import json
data = {'name': 'Xiao Ming', 'age': 18, 'city': 'Beijing'}
json_str = json.dumps(data, ensure_ascii=False)
print(json_str)
The ensure_ascii=False
parameter ensures Chinese characters display correctly instead of being converted to Unicode.
Practical Applications
With all this said, you might wonder: how are these modules used in real development? Let me give you a few examples.
Batch Rename Files
Suppose you have a bunch of photo files and want to rename them by shooting date, you can do this:
import os
from datetime import datetime
def rename_photos(directory):
for filename in os.listdir(directory):
if filename.endswith('.jpg'):
filepath = os.path.join(directory, filename)
timestamp = os.path.getmtime(filepath)
date = datetime.fromtimestamp(timestamp)
new_name = f"{date.strftime('%Y%m%d_%H%M%S')}.jpg"
os.rename(filepath, os.path.join(directory, new_name))
print(f"Renamed: {filename} -> {new_name}")
rename_photos('C:/Users/YourName/Pictures')
This script traverses all .jpg files in the specified directory, retrieves their modification time, and renames them using the format "YYYYMMDD_HHMMSS".
Simple Logger
If you want to add a logging feature to your program, you can create a simple logger using the datetime and os modules:
import os
from datetime import datetime
class SimpleLogger:
def __init__(self, log_dir):
self.log_dir = log_dir
if not os.path.exists(log_dir):
os.makedirs(log_dir)
def log(self, message):
timestamp = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
log_message = f"[{timestamp}] {message}
"
log_file = os.path.join(self.log_dir, f"{datetime.now().strftime('%Y%m%d')}.log")
with open(log_file, 'a', encoding='utf-8') as f:
f.write(log_message)
print(log_message.strip())
logger = SimpleLogger('logs')
logger.log("Program started")
logger.log("Performed an action")
logger.log("Program ended")
This logger creates log files named by date in the specified directory and prefixes each log entry with a timestamp.
Data Analysis Helper
Suppose you have a JSON format sales data file and want to quickly calculate the total sales, you can do this:
import json
from datetime import datetime
def analyze_sales(filename):
with open(filename, 'r', encoding='utf-8') as f:
data = json.load(f)
total_sales = sum(item['price'] * item['quantity'] for item in data)
date_sales = {}
for item in data:
date = datetime.fromisoformat(item['date']).date()
date_sales[date] = date_sales.get(date, 0) + item['price'] * item['quantity']
print(f"Total sales: ¥{total_sales:.2f}")
print("Daily sales:")
for date, sales in sorted(date_sales.items()):
print(f"{date}: ¥{sales:.2f}")
analyze_sales('sales_data.json')
This script reads a JSON file, calculates total sales, and summarizes sales data by date.
Conclusion
Through these examples, do you have a new understanding of the Python Standard Library? It not only simplifies daily programming tasks but also plays a crucial role in real projects. From file operations to data processing, from time management to random number generation, the Python Standard Library is almost omnipotent.
Of course, we've only scratched the surface here; there's still much treasure in the Python Standard Library waiting for you to discover. I suggest trying these modules more in your daily programming, and I'm sure you'll find more surprises.
Remember, using the Standard Library can make your code more concise, efficient, and professional. Next time you face a programming challenge, consider: is there a ready-made solution in the Standard Library?
Have you used the Python Standard Library in your projects? What interesting application scenarios have you encountered? Feel free to share your experiences and thoughts in the comments. Let's explore the infinite possibilities of the Python Standard Library together!
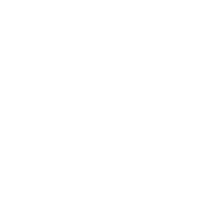
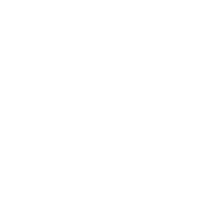