Have you ever felt that your Python code was missing something? Or found yourself writing a lot of repetitive code for common tasks? Don't worry, the Python Standard Library was created to solve these problems! Today, let's dive deep into the core application areas of the Python Standard Library and see how it can make your code more concise, efficient, and even elegant.
File Operations
When it comes to programming, file operations are one of the most basic and common tasks. Whether it's reading configuration files, processing logs, or saving data, we can't do without file operations. Python provides a simple yet powerful file operation interface that allows us to easily handle various file processing needs.
Basic Reading and Writing
First, let's start with the most basic file reading and writing. In Python, the open()
function is used to open files. This function is like a magic key that can open the doors to various files for us.
with open('example.txt', 'r') as file:
content = file.read()
print(content)
with open('output.txt', 'w') as file:
file.write("Hello, Python!")
You might ask, "Why use the with
statement?" Good question! Using the with
statement ensures that the file is automatically closed after use, which prevents resource leaks and is a good programming practice. I personally think it's like an automatic door closer - convenient and safe, so why not use it?
Advanced Techniques
Of course, file operations are far more than just simple reading and writing. Python also provides some advanced techniques that allow us to handle files more flexibly.
For example, you can use the seek()
method to move the cursor position in a file:
with open('example.txt', 'r+') as file:
file.seek(10) # Move to the 10th byte
print(file.read(5)) # Read 5 bytes starting from the 10th byte
Or you can use the os
module for more complex file operations:
import os
os.rename('old_name.txt', 'new_name.txt')
os.remove('unwanted_file.txt')
os.mkdir('new_directory')
These operations may seem simple, but they are frequently used in actual projects. I remember once I had to batch process hundreds of log files, and without these convenient functions, the workload would have been enormous!
Network Programming
In this internet age, the importance of network programming cannot be overstated. Whether building web applications or performing data scraping, Python's network programming capabilities can make you feel right at home.
Socket Programming
Socket is the foundation of network programming, and Python's socket
module provides a low-level network communication interface.
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect(('www.python.org', 80))
s.sendall(b'GET / HTTP/1.1\r
Host: www.python.org\r
\r
')
data = s.recv(1024)
print(data)
s.close()
This code might look a bit complex, but it's actually simulating the process of a browser accessing a webpage. Isn't it amazing? Through such low-level operations, we can better understand the principles of network communication.
HTTP Request Handling
Of course, if we just want to send HTTP requests, we have simpler options. The requests
library (although not a standard library, it has become a de facto standard) provides a higher-level HTTP client interface:
import requests
response = requests.get('https://api.github.com')
print(response.status_code)
print(response.json())
Look, we've completed an HTTP request with just a few lines of code! This is the charm of Python - simple yet powerful.
Data Processing and Analysis
In the era of big data, the importance of data processing and analysis goes without saying. Python can be said to be unrivaled in this field, mainly thanks to its powerful third-party library ecosystem.
NumPy Array Operations
NumPy is the cornerstone of Python data processing, providing high-performance multidimensional array objects and various mathematical functions.
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
print(arr * 2) # [2 4 6 8 10]
print(np.sin(arr)) # [0.84147098 0.90929743 0.14112001 -0.7568025 -0.95892427]
The power of NumPy lies in its ability to operate on entire arrays without the need for loops. This not only makes the code more concise but also greatly improves running efficiency. I remember being amazed by its speed when I first used NumPy!
Pandas DataFrame Usage
If NumPy is the cornerstone of data processing, then Pandas is the building erected on this foundation. Pandas provides powerful data structures and data analysis tools.
import pandas as pd
df = pd.DataFrame({
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Paris', 'London']
})
print(df[df['Age'] > 28])
print(df['Age'].mean())
Pandas' DataFrame is like a super spreadsheet. You can perform various operations on it, from simple filtering to complex statistical analysis. I once used Pandas to process a CSV file containing millions of rows of data, and the entire process was so smooth that it left me in awe.
Practical Application Cases
After discussing so much theory, let's see how these powerful tools are applied in actual projects!
File System Management
Suppose you need to batch rename all files in a directory, adding a timestamp prefix. This task may seem simple, but if there are many files, manual operation would be very time-consuming. Let's see how to easily implement this with Python:
import os
from datetime import datetime
def batch_rename(directory):
for filename in os.listdir(directory):
if os.path.isfile(os.path.join(directory, filename)):
timestamp = datetime.now().strftime("%Y%m%d_%H%M%S_")
new_filename = timestamp + filename
os.rename(os.path.join(directory, filename),
os.path.join(directory, new_filename))
print(f"Renamed {filename} to {new_filename}")
batch_rename("/path/to/your/directory")
This script adds a timestamp prefix to each file in the directory. Isn't it cool? Imagine if you had hundreds or thousands of files to rename, this script could save you a lot of time!
Network Application Development
Now, let's see how to quickly build a simple web server using Python. This example may look basic, but it actually demonstrates Python's powerful capabilities in network application development:
from http.server import HTTPServer, SimpleHTTPRequestHandler
import json
class SimpleJSONHandler(SimpleHTTPRequestHandler):
def do_GET(self):
self.send_response(200)
self.send_header('Content-type', 'application/json')
self.end_headers()
data = {
"message": "Hello, World!",
"path": self.path
}
self.wfile.write(json.dumps(data).encode())
httpd = HTTPServer(('localhost', 8000), SimpleJSONHandler)
print("Server running on http://localhost:8000")
httpd.serve_forever()
Run this code, and you'll have a simple JSON API server! It will return a JSON response to all GET requests, containing a greeting message and the requested path. This rapid prototyping capability is very useful in the early stages of development, allowing you to quickly validate ideas.
Data Science Project
Finally, let's look at a data science example. Suppose you have a CSV file containing sales data for a company, and you need to clean, analyze, and visualize this data. Here's a simple example:
import pandas as pd
import matplotlib.pyplot as plt
df = pd.read_csv('sales_data.csv')
df = df.dropna()
df['Date'] = pd.to_datetime(df['Date'])
monthly_sales = df.groupby(df['Date'].dt.to_period("M"))['Amount'].sum()
plt.figure(figsize=(12,6))
monthly_sales.plot(kind='bar')
plt.title('Monthly Sales')
plt.xlabel('Month')
plt.ylabel('Total Sales Amount')
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
This script reads sales data, performs simple cleaning and processing, and then creates a bar chart of monthly sales. In actual projects, you might need to perform more complex data processing and analysis, but this example demonstrates the basic workflow of Python in the field of data science.
Summary
Through these examples, we can see the powerful capabilities of the Python Standard Library (and some commonly used third-party libraries) in file operations, network programming, and data processing. These tools not only simplify our daily programming tasks but also help us build complex applications and perform in-depth data analysis.
Remember, these are just the tip of the iceberg. Python's ecosystem has countless treasures waiting for you to discover. I suggest you try using these libraries more in your daily programming, and you'll find they can greatly improve your work efficiency.
Finally, I want to say that programming is like an art, and Python and its standard library are our brushes and paints. Once you master these tools, you can create amazing works. So, keep exploring, keep learning, and let's create wonders together in the world of Python!
Do you have any interesting Python project experiences? Feel free to share your stories in the comments section, let's exchange ideas and grow together!
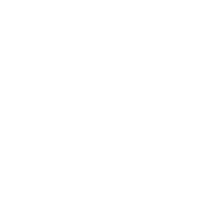
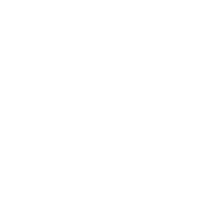