Hello, dear readers! Today, we are going to dive deep into the powerful and fascinating world of Python Object-Oriented Programming (OOP). As a Python enthusiast, I’ve always been drawn to the elegance and flexibility of OOP. Let’s uncover the mystery of OOP and see what makes it so magical!
Origin
I remember when I first started learning Python, I was very confused about the concept of OOP. I could understand functional programming, but objects, classes, and inheritance seemed like a foreign language to me. Then one day, I suddenly realized—OOP is essentially about simulating the structure of the real world! Since then, my understanding of OOP has skyrocketed. Today, I want to share my learning insights with you, hoping to help you grasp the essence of OOP more quickly.
Essence
So, what exactly is OOP? Simply put, OOP is a programming paradigm that packages data and the methods that process that data together. It views a program as a collection of objects, each containing data and methods to manipulate that data.
This idea aligns well with our perception of the real world. For example, we can view a student as an object with attributes like name and age (data), and behaviors like studying and taking exams (methods). Through OOP, we can express this structure more intuitively in code.
Comparison
To better understand the advantages of OOP, let’s first look at how traditional Procedural Programming works:
student_name = "Xiaoming"
student_age = 18
student_score = 95
def print_student_info(name, age, score):
print(f"Student {name}, Age {age}, Score {score}")
print_student_info(student_name, student_age, student_score)
In this approach, data and functions are separate. When we need to handle multiple students, the code becomes lengthy and difficult to maintain.
Now let’s see how to implement it using OOP:
class Student:
def __init__(self, name, age, score):
self.name = name
self.age = age
self.score = score
def print_info(self):
print(f"Student {self.name}, Age {self.age}, Score {self.score}")
xiaoming = Student("Xiaoming", 18, 95)
xiaoming.print_info()
You see, doesn’t it feel clearer and more organized? All data and methods related to the student are encapsulated in the Student
class. This is the charm of OOP!
Core
Next, let’s delve into the three core features of OOP: encapsulation, inheritance, and polymorphism. These concepts might sound abstract, but they all originate from our understanding of the real world.
Encapsulation
Encapsulation is like putting a protective layer on objects, exposing only the necessary interfaces for external access. It hides the internal details of the object, providing better data protection.
Let’s look at an example:
class BankAccount:
def __init__(self, owner, balance=0):
self.__owner = owner # Private attribute
self.__balance = balance # Private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
print(f"Deposit successful, current balance: {self.__balance}")
else:
print("Deposit amount must be greater than 0")
def withdraw(self, amount):
if 0 < amount <= self.__balance:
self.__balance -= amount
print(f"Withdrawal successful, current balance: {self.__balance}")
else:
print("Invalid withdrawal amount or insufficient balance")
def get_balance(self):
return self.__balance
account = BankAccount("Zhang San", 1000)
account.deposit(500) # Deposit successful, current balance: 1500
account.withdraw(2000) # Invalid withdrawal amount or insufficient balance
print(account.get_balance()) # 1500
In this example, we set the account balance __balance
as a private attribute, which cannot be directly accessed or modified from outside. It can only be operated through the deposit
and withdraw
methods, ensuring data security.
Inheritance
Inheritance allows us to create new classes based on existing ones. The new class (subclass) can inherit the attributes and methods of the parent class. This not only increases code reusability but also reflects hierarchical relationships between entities.
Here’s an example with students and teachers:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def introduce(self):
print(f"I am {self.name}, {self.age} years old")
class Student(Person):
def __init__(self, name, age, grade):
super().__init__(name, age)
self.grade = grade
def study(self):
print(f"{self.name} is studying")
class Teacher(Person):
def __init__(self, name, age, subject):
super().__init__(name, age)
self.subject = subject
def teach(self):
print(f"{self.name} is teaching {self.subject}")
student = Student("Xiaoming", 15, "Grade 3")
teacher = Teacher("Mr. Wang", 35, "Mathematics")
student.introduce() # I am Xiaoming, 15 years old
student.study() # Xiaoming is studying
teacher.introduce() # I am Mr. Wang, 35 years old
teacher.teach() # Mr. Wang is teaching Mathematics
In this example, both Student
and Teacher
inherit from the Person
class. They not only have the attributes and methods of Person
but also add specific attributes and methods of their own. Doesn’t this structure match our understanding of the real world?
Polymorphism
Polymorphism allows objects of different classes to respond to the same message. It provides code flexibility, allowing us to use a unified interface to operate on different types of objects.
Let’s look at an example of animal sounds:
class Animal:
def make_sound(self):
pass
class Dog(Animal):
def make_sound(self):
return "Woof!"
class Cat(Animal):
def make_sound(self):
return "Meow!"
class Duck(Animal):
def make_sound(self):
return "Quack!"
def animal_sound(animal):
print(animal.make_sound())
dog = Dog()
cat = Cat()
duck = Duck()
animal_sound(dog) # Woof!
animal_sound(cat) # Meow!
animal_sound(duck) # Quack!
In this example, the animal_sound
function can accept any Animal
type of object. Whether it’s a dog, cat, or duck, it can correctly produce the corresponding sound. This is the beauty of polymorphism—one interface, multiple implementations!
Advanced
After grasping the basic concepts of OOP, let’s look at some more advanced features. These features can make your code more flexible and powerful.
Special Methods (Magic Methods)
Python has some special methods whose names are surrounded by double underscores, such as __init__
, __str__
, __len__
, etc. These methods allow us to customize the behavior of objects.
Here’s an example:
class Book:
def __init__(self, title, author, pages):
self.title = title
self.author = author
self.pages = pages
def __str__(self):
return f"{self.title} by {self.author}"
def __len__(self):
return self.pages
def __eq__(self, other):
if not isinstance(other, Book):
return False
return self.title == other.title and self.author == other.author
book1 = Book("Python Study Notes", "Zhang San", 200)
book2 = Book("Python Study Notes", "Zhang San", 200)
book3 = Book("Java Introduction", "Li Si", 300)
print(book1) # Python Study Notes by Zhang San
print(len(book1)) # 200
print(book1 == book2) # True
print(book1 == book3) # False
In this example, we define the __str__
method to customize the string representation of the object, the __len__
method to define the length of the object, and the __eq__
method to define equality comparison of objects. These special methods make our Book
class more powerful and intuitive.
Class Methods and Static Methods
In addition to instance methods, Python supports class methods and static methods. Class methods can access and modify the class state, while static methods are like regular functions but reside in the class namespace.
Here’s an example:
class Pizza:
total_pizzas = 0
def __init__(self, size):
self.size = size
Pizza.total_pizzas += 1
@classmethod
def from_inch(cls, inch):
return cls(inch * 2.54)
@staticmethod
def is_valid_size(size):
return 10 <= size <= 50
@classmethod
def get_total_pizzas(cls):
return cls.total_pizzas
pizza1 = Pizza(30)
pizza2 = Pizza.from_inch(12) # Create object using class method
print(Pizza.is_valid_size(30)) # True
print(Pizza.is_valid_size(60)) # False
print(Pizza.get_total_pizzas()) # 2
In this example, from_inch
is a class method that creates a Pizza
object using inches. is_valid_size
is a static method used to check if the size is valid. get_total_pizzas
is another class method used to get the total number of pizzas created.
Property Decorators
Python provides the @property
decorator, which allows us to access methods like attributes. This not only simplifies the code but also provides better encapsulation.
Here’s an example:
class Circle:
def __init__(self, radius):
self._radius = radius
@property
def radius(self):
return self._radius
@radius.setter
def radius(self, value):
if value <= 0:
raise ValueError("Radius must be greater than 0")
self._radius = value
@property
def area(self):
return 3.14 * self._radius ** 2
circle = Circle(5)
print(circle.radius) # 5
print(circle.area) # 78.5
circle.radius = 10
print(circle.radius) # 10
print(circle.area) # 314.0
try:
circle.radius = -1
except ValueError as e:
print(e) # Radius must be greater than 0
In this example, we use @property
to turn the radius
and area
methods into properties. This way, we can use them like attributes while also performing value validation.
Application
After learning so many OOP concepts, you might ask: what’s the use of these in actual programming? Let me show you some applications of OOP in real projects.
Code Reuse and Modularity
One of the biggest advantages of OOP is increased code reusability and modularity. By creating general base classes, we can reuse code across multiple projects.
For example, suppose we are developing a game with various characters:
class Character:
def __init__(self, name, health, attack):
self.name = name
self.health = health
self.attack = attack
def is_alive(self):
return self.health > 0
def take_damage(self, damage):
self.health -= damage
if self.health < 0:
self.health = 0
def attack_target(self, target):
target.take_damage(self.attack)
class Warrior(Character):
def __init__(self, name):
super().__init__(name, health=100, attack=10)
def battle_cry(self):
print(f"{self.name}: For glory!")
class Mage(Character):
def __init__(self, name):
super().__init__(name, health=80, attack=15)
def cast_spell(self):
print(f"{self.name} casts a spell!")
warrior = Warrior("Arthur")
mage = Mage("Merlin")
warrior.attack_target(mage)
print(f"{mage.name}’s health: {mage.health}") # Merlin’s health: 70
mage.cast_spell() # Merlin casts a spell!
warrior.battle_cry() # Arthur: For glory!
By creating a general Character
class, we can easily add new character types without rewriting basic attributes and methods. This greatly improves code maintainability and extensibility.
Design Patterns
Another important application of OOP is implementing various design patterns. Design patterns are best practices for solving common programming problems. Let’s look at a simple Singleton pattern example:
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
def __init__(self):
self.data = []
def add_data(self, item):
self.data.append(item)
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # True
s1.add_data("Hello")
s2.add_data("World")
print(s1.data) # ['Hello', 'World']
print(s2.data) # ['Hello', 'World']
In this example, no matter how many times we create instances of the Singleton
class, they all point to the same object. This pattern is very useful when a globally unique object is needed, such as a configuration manager or a database connection pool.
Conclusion
Through this article, we have explored all aspects of Python Object-Oriented Programming. From basic concepts to advanced features, from theory to practice, we have walked the learning path of OOP together.
Let’s recap what we have learned: 1. Basic concepts of OOP: objects, classes, encapsulation, inheritance, and polymorphism 2. Definition and use of classes in Python 3. Application of special methods (magic methods) 4. Class methods and static methods 5. Use of property decorators 6. Application of OOP in real projects
I hope this article helps you better understand and apply OOP. Remember, the best way to learn programming is to practice. Try rewriting some of your old code using OOP, and you’ll find that the code becomes clearer and easier to maintain.
Do you have any questions about OOP? Or do you have any insights about using OOP that you want to share? Feel free to leave a comment, and let’s discuss and progress together!
The world of programming is vast and boundless, and OOP is just one exciting part of it. Stay curious, keep learning and practicing, and you will surely become an outstanding Python programmer. Good luck!
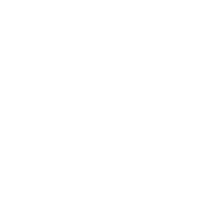
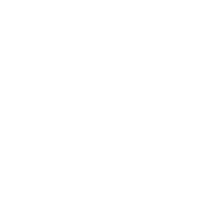
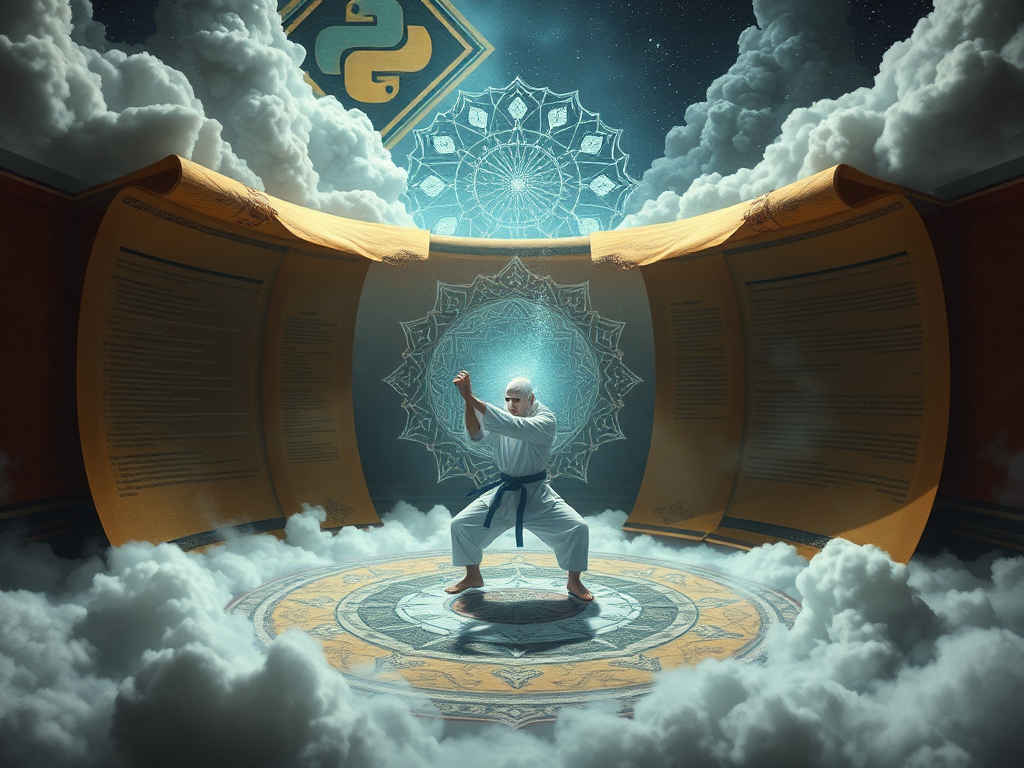
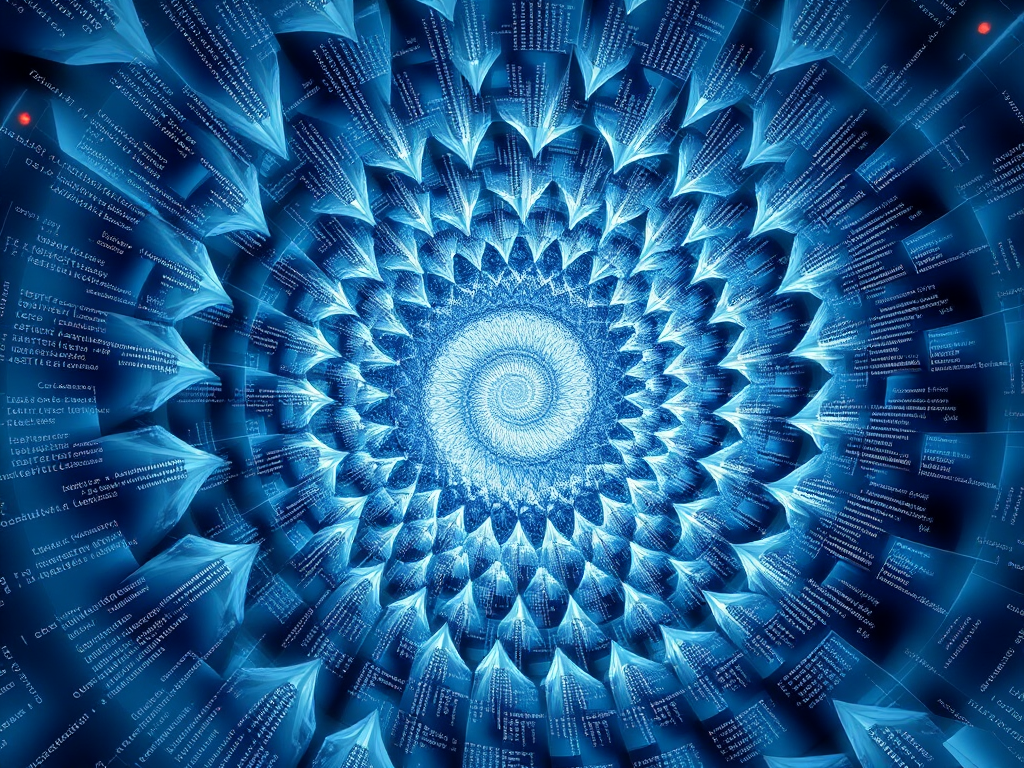
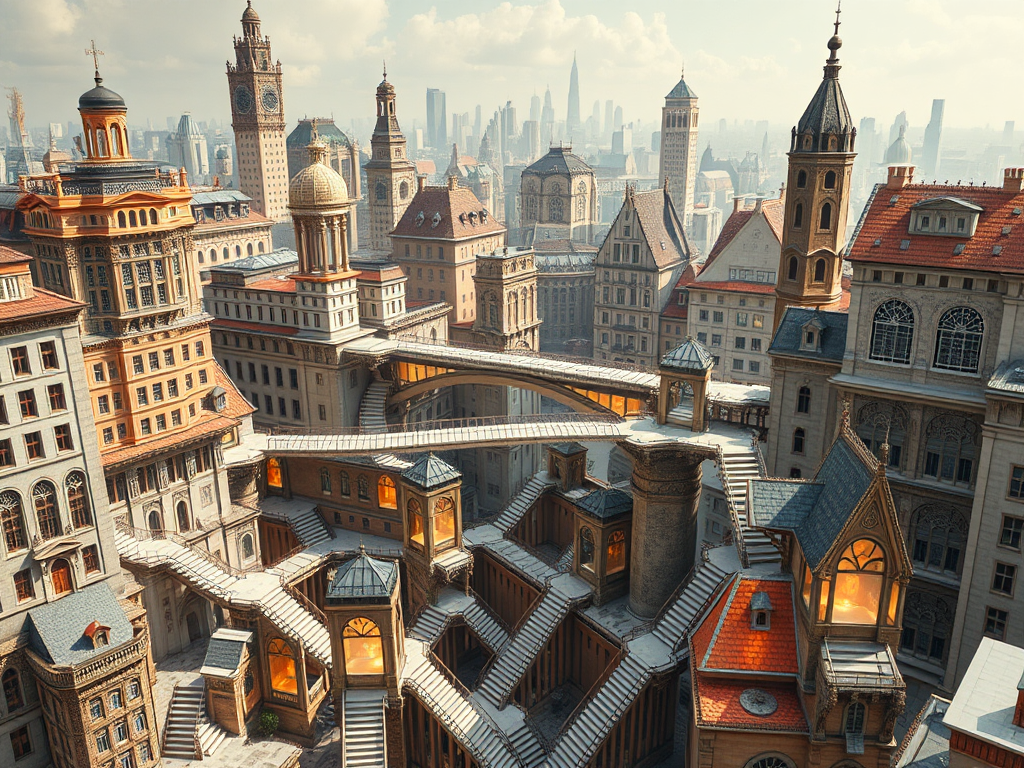